What follows are instructions on how to build a simple yet funny and scary countdown timer with an Arduino Nano and a 4 digit 7 segment LED display. Apart from the big bright red numbers, a small buzzer beeping in sync to the countdown will add to the dramatic effect…
Hardware:
- Arduino Nano (even a CH340 clone is good enough)
- 4 digit 7 segment display (based on the 74HC595)
- tiny 5V buzzer (optional)
Software:
//**************************************************************************//
// Name : countdown timer //
// Features: SPI 7seg-LED //
// Target : ATMEGA328P / Arduino //
// Clock : 16MHz //
// Author : Alex Scafidas //
// Date : 12 Dec 2021 //
// Ver : 1.00.000 //
// Storage : ProgMem> % Globalvar> % //
//**************************************************************************//
#define LED_DATA 11 //Pin connected to DS of 74HC595
#define LED_CLK 12 //Pin connected to SH_CP of 74HC595
#define LED_LATCH 13 //Pin connected to ST_CP of 74HC595
#define BEEPER 10
int secondsleft = 10;
void setup() {
pinMode(LED_LATCH, OUTPUT);
pinMode(LED_CLK, OUTPUT);
pinMode(LED_DATA, OUTPUT);
pinMode(BEEPER, OUTPUT);
}
void loop() {
while(secondsleft>0){
secondsleft -= 1;
seconds2LED(secondsleft);
secondsleft==0 ? theEnd() : beep();
}
}
void beep(){
digitalWrite(BEEPER,HIGH);
delay(200);
digitalWrite(BEEPER,LOW);
delay(800);
}
void theEnd(){
digitalWrite(BEEPER,HIGH);
delay(3000);
digitalWrite(BEEPER,LOW);
}
int getDigit(int number, int position){
int result = ((int)(number / pow(double(10),double(position))) % 10);
return result;
}
// 3 2 1 0
//[1][0][1][3]
void seconds2LED(int seconds){
int lednrcode[] = {0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90};
digitalWrite(LED_LATCH, LOW);
for(int digit=0;digit<4;digit++){
int num = getDigit(seconds,digit);
shiftOut(LED_DATA, LED_CLK, MSBFIRST, lednrcode[num]);
}
digitalWrite(LED_LATCH, HIGH);
}
Description:
Not much to say about this circuit. The code is self explanatory. Its a good opportunity to read upon shift registers. With every countdown all digits will be updated serially blazingly fast for the eye to see from least significant to most significant digit.
Schematic:
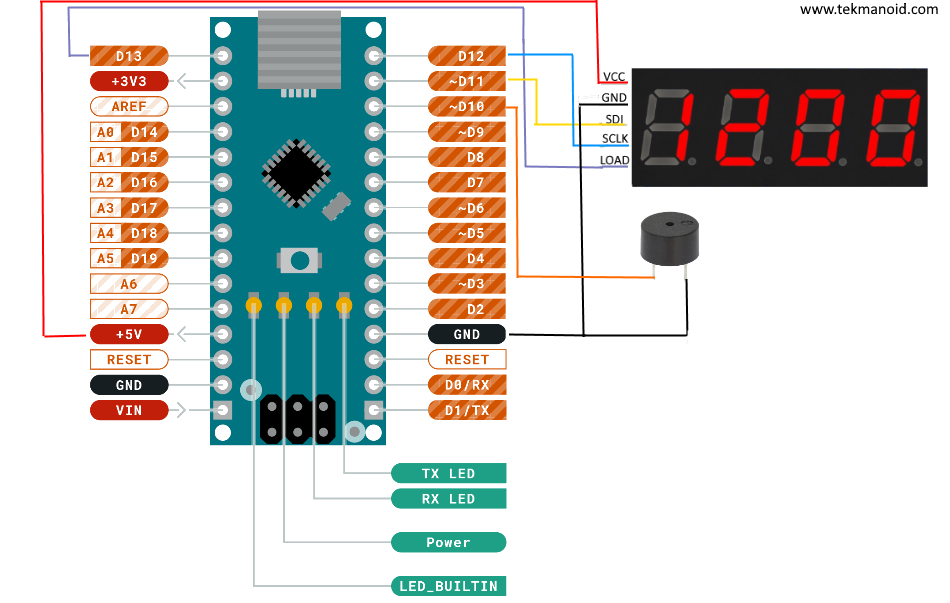